Common Spring Framework Mistakes developers should avoid
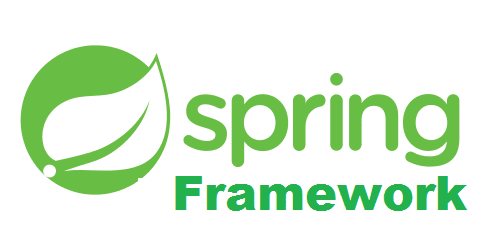
The Spring Framework is one of the most popular Java-based frameworks used by developers to develop web applications quickly and easily, leveraging powerful design patterns, such as Inversion of Control (IoC) and Dependency Injection (DI).
While these concepts can seem confusing to understand at first, developers who get started early with the framework learn to appreciate its powerful capabilities- and learn how to use it correctly. This article lists some common mistakes that developers make when using the Spring Framework and highlights how to avoid them.
Alert- Developers Should take Care of Important Things
Don’t use static imports
Static imports are often misused, leading to code that’s difficult to read and maintain. When using static imports, ensure that all the file code uses imported static members from the same class.
This will make your code more clear and maintainable. Also, don’t use too many static imports in a single file, as this can also lead to code that’s difficult to read.
Don’t check null in JdbcTemplate
When working with the JdbcTemplate class in Spring, there is no need to check for null values. This is because the JdbcTemplate will automatically handle this for you. If you try to check for null values, you may end up with a NullPointerException.
Don’t use deprecated methods in new projects.
When starting a new project, using the most up-to-date methods is essential. The Spring Framework constantly evolves, and deprecated methods are often removed in newer versions. This can cause your project to break if you’re not using the most recent framework version. Plus, using deprecated methods can make your code more difficult to read and maintain.
Lack of separation of concerns
In a monolithic application, there is a tight coupling between the data access layer, business logic layer, and presentation layer. This can lead to problems if you need to change one code area that affects another area.
For example, if you need to change the way data is accessed, you may also need to change the business logic and presentation layers as well. A better approach is to use separation of concerns, where each layer is independent of the others.
Reuse existing implementations
One of the most common mistakes made when using the Spring Framework is failing to reuse existing implementations. By doing so, you can avoid having to write unnecessary code and save time. Additionally, reusing current implementations can help improve the quality of your code.
Unable to accept dependency injection
One of the most common mistakes made when using the Spring Framework is failing to properly configure dependency injection. This can lead to all sorts of problems, from spring not being able to find your beans, to circular dependencies, and more. Fortunately, you can do a few things to avoid these problems.
Lack of test or improper test
One of the most common mistakes that developers make when working with the Spring Framework is a lack of test coverage, or improper testing. This can lead to critical bugs and vulnerabilities in your code.
Do not use annotation-based validation
Developers should not use annotation-based validation because it can lead to brittle code that is difficult to maintain. Plus, annotation-based validation can lead to false positives, which can cause problems down the road. It is important to consider all options before choosing annotation-based validation carefully.
Unable to Accept Dependency Injection
One of the most common mistakes made when using the Spring Framework is failing to configure dependency injection properly. This can lead to all sorts of problems, from code that fails to compile two classes that are not injected properly. By taking the time to properly configure dependency injection, you can avoid these problems and make sure that your code works as intended.
Internal structure “leaked”
The internal structure of the Spring Framework is complex and can be confusing for developers who are new to the framework. One common mistake is not understanding how the framework works and accidentally leaking information about the framework’s internals to the outside world.
This can lead to security vulnerabilities and other problems. To avoid this, it is crucial to understand how the framework works before using it. Another common mistake is using outdated or deprecated features of the Spring Framework.
Conclusion
If you’re new to the Spring framework, it’s important to be aware of the most common mistakes to avoid them in your projects. In this blog post, we’ve outlined 10 of the most common errors and provided some advice on how to prevent them for Java Spring Boot developer. By following these tips, you can help ensure that your Spring-based projects are successful.