10 Ways to Improve the Performance of Your ReactJS App
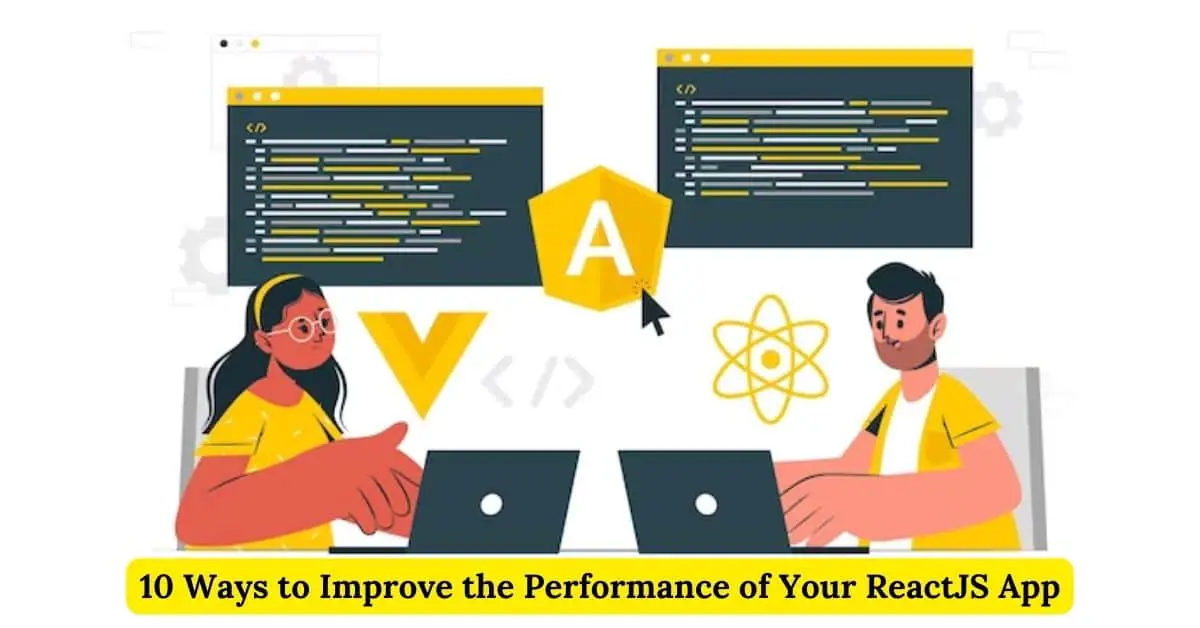
ReactJS is the dominant framework for building interactive, dynamic user interfaces in web development. With its component-based architecture and virtual DOM, ReactJS has gained immense popularity among developers. However, as applications grow in complexity and scale, maintaining optimal performance can become a challenge. To ensure that your ReactJS app delivers a seamless user experience, it’s crucial to focus on performance optimization. In this article, we will discuss ten effective ways for ReactJS developers to improve the performance of their applications.
Code Splitting
Code splitting is a technique that allows you to divide your application code into smaller, more manageable chunks. This way, you can load only the code that is necessary for the current user interaction, reducing the initial load time of your app. React provides a built-in solution for code splitting using dynamic imports. By using tools like Webpack, you can easily split your code into smaller bundles that are loaded on demand. This ensures faster initial page loads and improved overall performance.
To implement code splitting in your ReactJS app, consider using React’s React.lazy()
function and the Suspense
component. These features make it easier to load components asynchronously when they are needed.
Optimize Render Performance
React’s virtual DOM is designed to efficiently update the actual DOM, but inefficient rendering can still lead to performance issues. To optimize render performance, follow these guidelines:
- Use the
shouldComponentUpdate
orReact.memo
to prevent unnecessary renders. - Implement PureComponent or use
React.memo
it for functional components to prevent re-rendering when props or states haven’t changed. - Minimize the use of inline arrow functions in render methods, as they can create new function instances on each render.
By ensuring that your components update only when necessary, you can significantly improve the overall performance of your ReactJS app.
Use PureComponent and Memoization
React provides PureComponent
the React.memo
higher-order component for optimizing rendering. These tools help you avoid unnecessary re-renders by performing a shallow comparison of props and state before deciding whether a component should be updated.
For class components, PureComponent
automatically implements shouldComponentUpdate
a shallow prop and state comparison. Functional components React.memo
can be used to achieve the same effect. Utilizing these tools ensures that your components only re-render when their data has actually changed, leading to better performance.
Virtualize Long Lists
When rendering long lists or tables, it’s important to consider the impact on performance. Rendering a large number of items at once can lead to slow initial load times and reduced user experience. To address this, you can implement virtualization techniques, such as the react-virtualized
library or the built-in React window
.
Virtualization allows you to render only the visible items in the list, significantly reducing the amount of DOM manipulation required. This results in faster rendering and a smoother user interface.
Optimize Images and Media
Images and media files are often the largest assets in a web application. To improve performance, consider the following strategies:
- Use responsive images and the
srcset
attribute to serve different image sizes based on the device’s screen resolution. - Compress and optimize images before adding them to your project.
- Lazy load images to defer their loading until they come into the user’s viewport.
- Utilize image formats like WebP, which offer better compression and quality compared to older formats like JPEG and PNG.
Minimize Redux Usage
While Redux is a powerful state management library for React, excessive use of Redux can lead to performance bottlenecks. Every action dispatch in Redux triggers a state change and potentially causes component re-renders, even if the data hasn’t changed.
To improve performance, carefully assess whether every piece of data in your app needs to be managed by Redux. Local component states or contexts may be more efficient for managing certain types of data. Additionally, consider using the Redux Toolkit, which includes performance optimizations like memoization and batched updates.
Bundle Splitting and Lazy Loading
Bundle splitting is a technique that involves breaking your application code into smaller bundles or chunks. These bundles can then be loaded asynchronously as needed, reducing the initial load time of your app. Webpack, a popular bundler for React projects, provides excellent support for bundle splitting.
Lazy loading is closely related to bundle splitting and is achieved by using dynamic imports and React’s React.lazy()
function. With lazy loading, you can load parts of your application on-demand, improving the overall performance by reducing the initial download size.
Optimize Component Lifecycle
Understanding the React component lifecycle is crucial for optimizing performance. Make use of lifecycle methods like componentDidMount
, componentDidUpdate
, and componentWillUnmount
to manage side effects, such as data fetching, event listeners, or cleanup.
For modern React apps, consider using React hooks useEffect
to achieve the same functionality as lifecycle methods. Hooks provide a cleaner and more efficient way to manage component side effects and state changes.
Server-Side Rendering (SSR) and Pre-rendering
Server-side rendering (SSR) and pre-rendering are techniques that can significantly improve the initial load time of your React app. SSR involves rendering React components on the server and sending the pre-rendered HTML to the client, which can be especially beneficial for SEO and performance.
Next.js, a popular framework built on top of React, simplifies the implementation of SSR and pre-rendering. It provides features like automatic code splitting, route-based rendering, and static site generation (SSG) to optimize the performance of your app.
Profile and Optimize
Regularly profiling your ReactJS app is essential for identifying performance bottlenecks. Tools like the React Profiler and browser developer tools can help you pinpoint areas that need improvement. Once you’ve identified performance issues, use optimization techniques like memoization, lazy loading, and code splitting to address them.
Conclusion
As a ReactJS developer, optimizing the performance of your web applications is crucial for providing users with a fast and responsive experience. By implementing code splitting, optimizing rendering, virtualizing long lists, and following best practices, you can ensure that your ReactJS app runs smoothly and efficiently. Additionally, staying up-to-date with the latest developments in the React ecosystem and regularly profiling your app for performance bottlenecks will help you deliver high-quality applications that meet the demands of modern web users.
Read also: How to Hire the Best Web Developer in India for Your Project